Welcome to the ultimate resource for mastering Java Basics interview questions. Whether you’re a seasoned Java developer or just starting your journey, this guide is designed to equip you with in-depth knowledge and practical insights. We’ve curated a comprehensive list of interview questions, complete with expert sample answers and guidance on how to respond effectively. Plus, you can conveniently download the PDF version for offline learning. Let’s dive into the world of Java and sharpen your skills to ace your next interview.
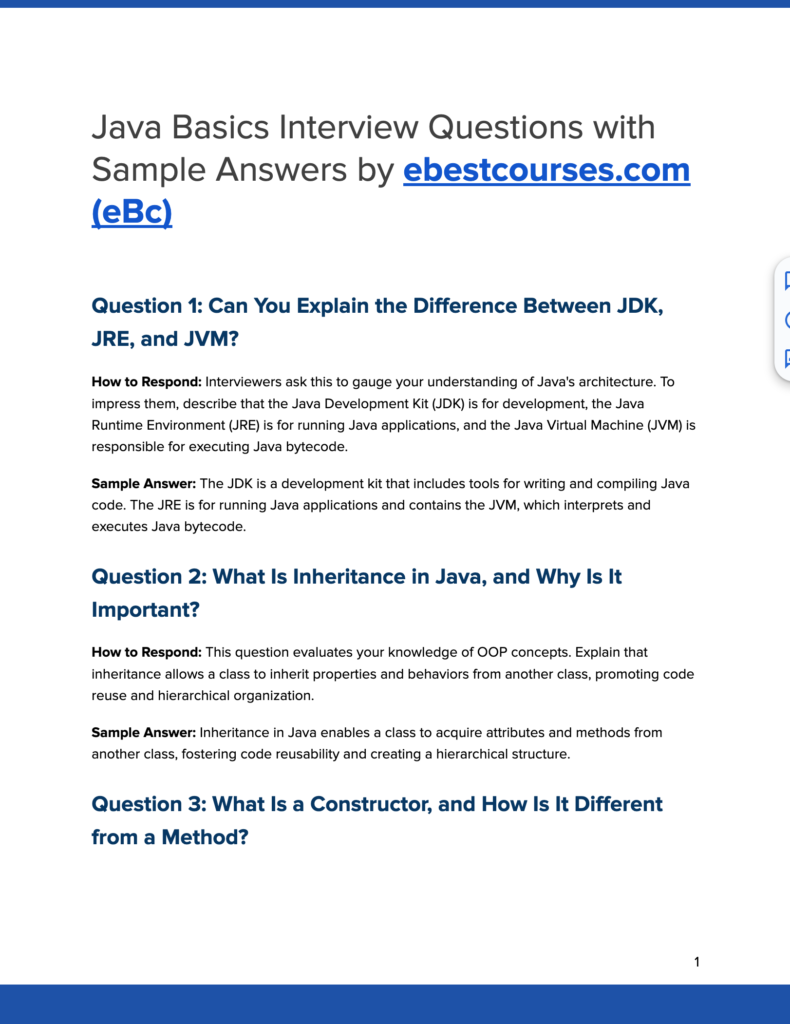
Download Java Basics Interview Questions with Sample Answers PDF
Enhance your Java skills with our downloadable PDF. Get ready for interviews with sample answers to common Java Basics questions. Download now for offline access.
Question 1: Can You Explain the Difference Between JDK, JRE, and JVM?
How to Respond: Interviewers ask this to gauge your understanding of Java’s architecture. To impress them, describe that the Java Development Kit (JDK) is for development, the Java Runtime Environment (JRE) is for running Java applications, and the Java Virtual Machine (JVM) is responsible for executing Java bytecode.
Sample Answer: The JDK is a development kit that includes tools for writing and compiling Java code. The JRE is for running Java applications and contains the JVM, which interprets and executes Java bytecode.
Question 2: What Is Inheritance in Java, and Why Is It Important?
How to Respond: This question evaluates your knowledge of OOP concepts. Explain that inheritance allows a class to inherit properties and behaviors from another class, promoting code reuse and hierarchical organization.
Sample Answer: Inheritance in Java enables a class to acquire attributes and methods from another class, fostering code reusability and creating a hierarchical structure.
Question 3: What Is a Constructor, and How Is It Different from a Method?
How to Respond: Showcase your grasp of fundamental Java concepts. Highlight that a constructor initializes objects, has the same name as the class, and lacks a return type, distinguishing it from methods.
Sample Answer: A constructor initializes objects, shares the class name, and doesn’t have a return type, in contrast to methods that perform specific tasks.
Question 4: Explain the ‘public static void main(String[] args)’ Method. Why Is It Essential?
How to Respond: This assesses your understanding of the main method, the entry point in Java. Elaborate that it’s public, static, void (no return value), and takes an array of strings as arguments.
Sample Answer: ‘public static void main(String[] args)’ is Java’s entry point, signifying that it’s accessible, doesn’t return a value, and accepts string arguments for command-line input.
Question 5: What Are Java Generics, and How Do They Enhance Code Quality?
How to Respond: Emphasize your grasp of generics’ role in type safety. Mention that they enable parameterized types, enhancing code reusability and quality by preventing type-related errors.
Sample Answer: Java generics provide type safety by introducing parameterized types, which enhance code reusability and quality by catching type-related issues at compile-time.
Question 6: Explain the ‘final’ Keyword in Java and Its Applications.
How to Respond: Showcase your knowledge of the ‘final’ keyword. Describe that it makes variables, methods, or classes unmodifiable, and detail its use in creating constants and preventing method overriding.
Sample Answer: In Java, ‘final’ makes variables, methods, or classes unalterable. It’s used for constants and ensures method overriding prevention.
Question 7: What Is Polymorphism, and How Does It Benefit Java Development?
How to Respond: Highlight your understanding of polymorphism. Explain it as the ability of objects to take on different forms, enabling flexibility, extensibility, and simpler code.
Sample Answer: Polymorphism allows objects to have multiple forms in Java, enhancing code flexibility, extensibility, and simplicity.
Question 8: Can You Explain Checked and Unchecked Exceptions in Java and Provide Examples?
How to Respond: Demonstrate your knowledge of exceptions. Distinguish checked exceptions (compile-time) and unchecked exceptions (runtime) and give examples for both.
Sample Answer: Checked exceptions are verified at compile-time, like IOException. Unchecked exceptions, e.g., NullPointerException, occur at runtime.
Question 9: How Does Java Support Multithreading, and What Is Synchronization?
How to Respond: Show your understanding of Java’s multithreading capabilities. Explain that Java allows concurrent execution, and synchronization is used to ensure thread safety by preventing multiple threads from accessing critical sections simultaneously.
Sample Answer: Java supports multithreading, enabling concurrent execution. Synchronization ensures thread safety by preventing simultaneous access to critical sections.
Question 10: What Are Java Annotations, and How Are They Used in Code?
How to Respond: Discuss your familiarity with annotations. Mention that they provide metadata to Java code, making it more informative and structured, and give examples like ‘@Override’ and ‘@Deprecated.’
Sample Answer: Java annotations offer metadata to code, enhancing its readability and structure. For instance, ‘@Override’ indicates method overriding, while ‘@Deprecated’ marks deprecated elements.
Question 11: Explain the Difference Between ‘ArrayList’ and ‘LinkedList’ in Java Collections.
How to Respond: Showcase your knowledge of Java collections. Describe that an ‘ArrayList’ is an array-based list, while a ‘LinkedList’ is a doubly-linked list. Explain their strengths and weaknesses.
Sample Answer: An ‘ArrayList’ in Java is an array-based list with dynamic sizing, while a ‘LinkedList’ is a doubly-linked list. ArrayLists are efficient for random access, whereas LinkedLists excel in insertions and deletions.
Question 12: What Is the Purpose of the ‘hashCode’ and ‘equals’ Methods in Java, and How Do They Work Together?
How to Respond: Highlight your understanding of ‘hashCode’ and ‘equals’ methods. Explain that ‘hashCode’ generates a unique code for an object, and ‘equals’ compares objects for equality. Describe their collaboration for proper data storage in collections.
Sample Answer: The ‘hashCode’ method generates a unique code for objects, and ‘equals’ compares objects for equality. These methods work together to ensure proper data storage and retrieval in collections.
Question 13: Can You Explain the ‘static’ Keyword in Java and Its Usage?
How to Respond: Elaborate on the ‘static’ keyword. Describe its role in creating class-level variables and methods that can be accessed without creating instances of the class.
Sample Answer: In Java, the ‘static’ keyword is used to create class-level variables and methods, enabling access without instantiating the class.
Question 14: What Are Anonymous Inner Classes in Java, and How Are They Implemented?
How to Respond: Showcase your knowledge of inner classes. Explain that anonymous inner classes are unnamed classes created within methods or code blocks. Describe their common use cases, like event handling.
Sample Answer: Anonymous inner classes in Java are unnamed classes defined within methods. They are often used for event handling and implementing interfaces.
Question 15: Can You Describe the Java Memory Model and How It Ensures Thread Safety?
How to Respond: Demonstrate your understanding of the Java Memory Model. Explain how it guarantees thread safety through concepts like ‘volatile’ and ‘synchronized.’
Sample Answer: The Java Memory Model ensures thread safety through ‘volatile’ and ‘synchronized’ keywords, allowing proper visibility and synchronization among threads.
Question 16: What Is the Role of the ‘super’ Keyword in Java Inheritance, and How Does It Work?
How to Respond: Explain the ‘super’ keyword in Java. Describe its use for invoking the superclass’s constructor or methods and how it prevents ambiguity in overridden methods.
Sample Answer: The ‘super’ keyword is used to access the superclass’s constructor or methods. It resolves ambiguity in overridden methods and ensures correct method execution.
Question 17: Describe the Principles of Java Garbage Collection and Its Importance.
How to Respond: Illustrate your knowledge of garbage collection in Java. Explain its purpose in reclaiming memory by identifying and collecting unreferenced objects.
Sample Answer: Java garbage collection reclaims memory by identifying and collecting unreferenced objects. It ensures efficient memory usage and prevents memory leaks.
Question 18: What Is the Purpose of the ‘this’ Keyword in Java, and How Is It Used for Handling Name Conflicts? Provide Examples.
How to Respond: Interviewers ask this to assess your knowledge of the ‘this’ keyword. Explain that ‘this’ is used to reference the current object and resolve name conflicts, providing examples.
Sample Answer: In Java, the ‘this’ keyword is used to reference the current object. It resolves name conflicts between instance variables and method parameters or for calling constructors within constructors.
Question 19: Explain the ‘try-with-resources’ Statement in Java and Its Benefits in Resource Management.
How to Respond: Show your knowledge of resource management in Java. Describe how ‘try-with-resources’ simplifies resource handling by automatically closing resources like files or sockets.
Sample Answer: The ‘try-with-resources’ statement in Java simplifies resource management by automatically closing resources, preventing resource leaks and ensuring efficient handling.
Question 20: Can You Describe Java’s ‘var’ Keyword and Its Role in Type Inference?
How to Respond: Discuss your familiarity with the ‘var’ keyword. Explain its use in local variable type inference, making code more concise and readable.
Sample Answer: Java’s ‘var’ keyword is used for local variable type inference, reducing verbosity and enhancing code readability by inferring variable types.
Question 21: What Are Annotations in Java, and How Are They Used for Metadata?
How to Respond: Highlight your understanding of Java annotations. Explain their role in providing metadata to classes, methods, or fields and give examples like ‘@Override’ and ‘@SuppressWarnings.’
Sample Answer: Java annotations offer metadata to code, enhancing its structure and providing information about classes, methods, or fields. For instance, ‘@Override’ signifies method overriding, while ‘@SuppressWarnings’ suppresses warnings.
Question 22: Explain the Concept of Exception Chaining in Java and Its Use Cases.
How to Respond: Describe exception chaining in Java. Explain how it helps preserve the original exception while adding contextual information. Provide examples of when and why it’s useful.
Sample Answer: Exception chaining in Java is the practice of preserving the original exception while adding context-specific information. It’s valuable when propagating errors across layers or modules, aiding in debugging and problem identification.
Question 23: Can You Differentiate Between Method Overloading and Method Overriding in Java?
How to Respond: Showcase your knowledge of method overloading and method overriding. Explain that overloading involves multiple methods with the same name but different parameters, while overriding pertains to a subclass providing its implementation for a superclass method.
Sample Answer: In Java, method overloading means having multiple methods with the same name but different parameters, while method overriding occurs when a subclass provides its implementation for a method inherited from a superclass.
Question 24: What Is Serialization in Java, and How Is It Implemented for Object Persistence?
How to Respond: Explain your understanding of object serialization in Java. Describe how it converts objects into byte streams for storage or transmission and mention the ‘Serializable’ interface.
Sample Answer: Serialization in Java is the process of converting objects into byte streams for storage or transmission. It’s implemented by implementing the ‘Serializable’ interface and using ObjectOutputStream for writing and ObjectInputStream for reading.
Question 25: Describe the ‘break’ and ‘continue’ Statements in Java and Their Applications in Loop Control.
How to Respond: Discuss the ‘break’ and ‘continue’ statements in Java. Explain that ‘break’ is used to exit loops prematurely, while ‘continue’ skips the current iteration and continues with the next.
Sample Answer: In Java, the ‘break’ statement is used to exit loops prematurely, while ‘continue’ skips the current iteration and proceeds with the next one.
These questions delve into more advanced Java concepts, including resource management, type inference, annotations, and exception handling. If you have specific areas or topics you’d like to focus on, feel free to let me know.
Question 26: What Is the Purpose of the ‘transient’ Keyword in Java, and When Would You Use It?
How to Respond: Explain your knowledge of the ‘transient’ keyword. Describe its role in marking variables as non-serializable and provide scenarios where it’s beneficial.
Sample Answer: The ‘transient’ keyword in Java designates variables as non-serializable, making them excluded from the serialization process. It’s valuable for fields that should not be persisted, such as caching or temporary data.
Question 27: Can You Explain the Concept of Lambda Expressions in Java and Their Use in Functional Programming?
How to Respond: Discuss your familiarity with lambda expressions. Describe their role in enabling functional programming by simplifying the creation of anonymous functions.
Sample Answer: Lambda expressions in Java allow the creation of anonymous functions, promoting functional programming by simplifying the definition of small, single-use methods, enhancing code readability.
Question 28: What Is a Java Thread, and How Do You Create and Manage Threads in a Java Program?
How to Respond: Showcase your understanding of Java threads. Explain that a thread is a lightweight process, and detail the creation and management of threads using ‘Thread’ or the ‘Runnable’ interface.
Sample Answer: A Java thread is a lightweight process that can run concurrently. Threads are created by extending the ‘Thread’ class or implementing the ‘Runnable’ interface. They can be managed through methods like ‘start,’ ‘join,’ and ‘sleep.’
Question 29: What Is Java’s ‘assert’ Statement, and How Does It Help in Debugging and Testing?
How to Respond: Explain the ‘assert’ statement in Java. Describe its use for testing and debugging by verifying specific conditions during program execution.
Sample Answer: The ‘assert’ statement in Java is used for testing and debugging by checking specified conditions and generating an error if they are false. It helps identify issues and validate assumptions.
Question 30: Can You Describe the Java ‘switch’ Statement and Its Differences Compared to ‘if-else’ Statements?
How to Respond: Discuss your knowledge of the ‘switch’ statement. Explain its use for multiple conditional branches and highlight the differences compared to ‘if-else’ statements.
Sample Answer: In Java, the ‘switch’ statement allows multiple conditional branches based on the value of an expression. It differs from ‘if-else’ statements by providing a more concise structure for handling multiple conditions.
Question 31: How Does the ‘finally’ Block Work in Java Exception Handling, and When Is It Useful?
How to Respond: Describe the ‘finally’ block in Java. Explain that it is executed regardless of whether an exception is thrown or not, and provide examples of its utility in resource cleanup.
Sample Answer: The ‘finally’ block in Java exception handling is executed irrespective of whether an exception is thrown or not. It’s beneficial for resource cleanup and ensures critical actions are performed before exiting a method or block.
Question 32: What Is Java’s ‘enum’ and Its Role in Defining Enumerations?
How to Respond: Explain your knowledge of ‘enum’ in Java. Describe it as a data type for defining enumerations and its use in representing a fixed set of values.
Sample Answer: In Java, an ‘enum’ is a data type used to define enumerations. It’s employed to represent a fixed set of named values, making code more readable and maintainable.
Question 33: How Do You Prevent Deadlocks in Java Multi-Threading, and What Strategies Can Be Applied?
How to Respond: Discuss your understanding of preventing deadlocks in Java. Explain techniques like acquiring locks in a consistent order, using timeouts, and employing higher-level concurrency utilities.
Sample Answer: Deadlocks in Java can be avoided by acquiring locks in a consistent order, setting timeouts, or utilizing higher-level concurrency utilities like ‘java.util.concurrent’ classes. These strategies ensure smoother multi-threading operation.
Question 34: What Is the Role of the ‘NIO’ (New I/O) Package in Java, and How Does It Improve I/O Operations?
How to Respond: Explain the significance of the ‘NIO’ package in Java. Describe its enhanced I/O capabilities, such as non-blocking operations and buffers, and how it improves efficiency.
Sample Answer: The ‘NIO’ (New I/O) package in Java introduces advanced I/O operations, including non-blocking I/O and memory-mapped buffers. It enhances efficiency by reducing resource consumption and supporting asynchronous I/O.
Question 35: Can You Explain Java’s ‘StringBuilder’ and ‘StringBuffer’ Classes, and How Are They Different from ‘String’?
How to Respond: Showcase your knowledge of ‘StringBuilder’ and ‘StringBuffer’ classes. Explain their roles in mutable string operations and compare them to immutable ‘String’ objects.
Sample Answer: ‘StringBuilder’ and ‘StringBuffer’ in Java are classes used for mutable string operations, allowing dynamic content modification. Unlike ‘String’ objects, they are not immutable and are suitable for situations where string content changes frequently.
Question 36: What Are Annotations in Java, and How Are They Used for Metadata?
Sample Answer: Java annotations offer metadata to code, enhancing its structure and providing information about classes, methods, or fields. For instance, ‘@Override’ signifies method overriding, while ‘@SuppressWarnings’ suppresses warnings.
How to Respond: Discuss your familiarity with Java annotations. Explain their role in providing metadata to classes, methods, or fields, and provide examples like ‘@Override’ and ‘@SuppressWarnings.’
Question 37: Explain the Principles of the ‘Model-View-Controller’ (MVC) Design Pattern in Java, and How Does It Facilitate Software Development?
How to Respond: Describe your knowledge of the ‘Model-View-Controller’ (MVC) design pattern in Java. Explain its separation of concerns and how it promotes modularity and maintainability in software development.
Sample Answer: The ‘Model-View-Controller’ (MVC) design pattern in Java divides software into three components: Model (data), View (user interface), and Controller (interaction logic). It separates concerns, enhancing modularity and maintainability in software development.
Question 38: Can You Describe the Concept of ‘Garbage Collection’ in Java and Its Role in Memory Management?
How to Respond: Explain your understanding of garbage collection in Java. Describe its responsibility for automatic memory management by reclaiming unreferenced objects.
Sample Answer: Garbage collection in Java is the automatic process of reclaiming memory by identifying and collecting objects that are no longer referenced. It ensures efficient memory management and prevents memory leaks.
Question 39: What Is ‘Dependency Injection’ in Java, and How Does It Enhance Code Modularity and Testing?
How to Respond: Discuss your knowledge of ‘Dependency Injection’ in Java. Explain its role in enhancing code modularity, maintainability, and testability by decoupling components.
Sample Answer: ‘Dependency Injection’ in Java is a design pattern that promotes code modularity, maintainability, and testability by decoupling components and allowing the injection of dependencies from external sources.
Question 40: How Do You Handle Concurrent Modification in Java Collections, and What Strategies Can Be Applied?
How to Respond: Demonstrate your understanding of concurrent modification issues in Java collections. Explain strategies like using ‘Iterator’ and concurrent collection classes to prevent exceptions.
Sample Answer: To handle concurrent modification in Java collections, strategies include using ‘Iterator’ for iteration and employing concurrent collection classes such as ‘ConcurrentHashMap’ to prevent exceptions and ensure thread safety.
Question 41: What Is the Purpose of the ‘volatile’ Keyword in Java, and How Does It Ensure Thread Visibility and Synchronization?
How to Respond: Explain your understanding of the ‘volatile’ keyword. Describe its role in ensuring thread visibility by making variables accessible by multiple threads and preventing caching.
Sample Answer: The ‘volatile’ keyword in Java guarantees thread visibility by making variables accessible by multiple threads. It prevents variable caching and ensures synchronized access among threads.
Question 42: Can You Explain the Concept of ‘Recursion’ in Java and Provide an Example of a Recursive Function?
How to Respond: Discuss your knowledge of recursion in Java. Explain its use in solving problems by breaking them into smaller, identical subproblems, and provide an example of a recursive function.
Sample Answer: Recursion in Java is a technique where a function calls itself to solve a problem by dividing it into smaller, identical subproblems. An example is the calculation of factorial, where ‘n!’ is computed by multiplying ‘n’ with ‘(n-1)!’.
Question 43: What Are Java Annotations, and How Can Custom Annotations Be Created and Used?
How to Respond: Highlight your understanding of Java annotations. Describe their role in providing metadata and explain how custom annotations can be defined and utilized.
Sample Answer: Java annotations offer metadata to code, making it more structured. Custom annotations can be created by defining new annotation types and then applied to classes, methods, or fields to convey additional information or instructions.
Question 44: Explain the ‘try-catch-finally’ Block in Java Exception Handling, and How Does It Ensure Exception Cleanup and Handling?
How to Respond: Describe the ‘try-catch-finally’ block in Java. Explain that it ensures proper resource cleanup and exception handling by executing code within the ‘finally’ block regardless of exceptions.
Sample Answer: The ‘try-catch-finally’ block in Java is used for exception handling and guarantees proper resource cleanup. The ‘finally’ block ensures that certain code is executed, regardless of whether exceptions occur or not.
Question 45: What Are Java Collections, and How Do You Choose the Right Collection Type for Specific Use Cases?
How to Respond: Explain your knowledge of Java collections. Describe the various collection types like lists, sets, and maps, and explain how to select the appropriate collection for specific scenarios.
Sample Answer: Java collections are data structures that store and manipulate groups of objects. Selecting the right collection type depends on the specific use case. Lists are suitable for ordered data, sets for unique values, and maps for key-value associations.
Question 46: Can You Explain the Concept of ‘Polymorphism’ in Java and Provide an Example of Its Application?
How to Respond: Discuss your understanding of polymorphism in Java. Explain it as the ability of objects to take on different forms, and provide an example, such as using inheritance and method overriding.
Sample Answer: Polymorphism in Java enables objects to have multiple forms. For instance, a base class reference can point to derived class objects, and method overriding demonstrates polymorphic behavior.
Question 47: Describe the ‘equals’ and ‘hashCode’ Methods in Java, Their Relationship, and How to Implement Them for Proper Object Comparison and Hashing.
How to Respond: Highlight your knowledge of the ‘equals’ and ‘hashCode’ methods. Explain that ‘equals’ compares object equality, while ‘hashCode’ generates hash codes, and describe their relationship for correct object comparison and hashing.
Sample Answer: In Java, the ‘equals’ method compares object equality, and ‘hashCode’ generates hash codes. These methods are related, as objects that are considered equal must have the same hash code. Implementing them correctly is crucial for proper object comparison and hashing.
Question 48: What Is ‘Method Overloading’ in Java, and How Does It Work? Provide Examples of Overloaded Methods.
How to Respond: Explain your understanding of method overloading in Java. Describe it as the practice of having multiple methods with the same name but different parameters, and provide examples of overloaded methods.
Sample Answer: Method overloading in Java involves having multiple methods with the same name but different parameters. For example, a class can have multiple constructors with varying parameter lists.
Question 49: Can You Explain the Concept of ‘Concurrency’ and ‘Parallelism’ in Java, and How Do They Relate to Multithreading?
How to Respond: Discuss your knowledge of concurrency and parallelism in Java. Explain that concurrency deals with the execution of multiple tasks at the same time, while parallelism is the simultaneous execution of tasks on multiple processors. Relate these concepts to multithreading.
Sample Answer: Concurrency in Java involves managing the execution of multiple tasks, while parallelism is the simultaneous execution of tasks on multiple processors. In multithreading, concurrency is achieved by having multiple threads running, while parallelism occurs when threads run on multiple processors to enhance performance.
Question 50: What Is ‘Exception Handling’ in Java, and How Does It Improve the Robustness of Code? Provide Examples of Exception Types.
How to Respond: Explain your understanding of exception handling in Java. Describe it as the practice of dealing with errors or exceptional situations, improving code robustness. Provide examples of common exception types like ‘NullPointerException’ and ‘IOException.’
Sample Answer: Exception handling in Java is the practice of managing errors or exceptional situations to improve code robustness. Common exception types include ‘NullPointerException’ when trying to access a null reference and ‘IOException’ when dealing with input/output operations.
Question 51: Explain the ‘super’ Keyword in Java Inheritance and How It Resolves Ambiguity in Overridden Methods. Provide an Example.
How to Respond: Describe the ‘super’ keyword in Java inheritance. Explain its use for invoking superclass constructors or methods and how it resolves ambiguity in overridden methods. Provide a practical example.
Sample Answer: In Java inheritance, the ‘super’ keyword is used to access superclass constructors or methods. It resolves ambiguity in overridden methods by specifying which method to call. For example, ‘super.method()’ would call the superclass’s method.
Question 52: What Is ‘Abstract Class’ in Java, and How Does It Differ from an Interface?
How to Respond: Discuss your knowledge of abstract classes in Java. Explain them as classes that cannot be instantiated and may contain abstract methods. Highlight the differences between abstract classes and interfaces.
Sample Answer: In Java, an abstract class is a class that cannot be instantiated and may include abstract methods. The key difference from an interface is that an abstract class can have method implementations, while interfaces only declare method signatures.
Question 53: Can You Explain the ‘finalize’ Method in Java and How It Relates to Object Cleanup and Resource Reclamation?
How to Respond: Describe the ‘finalize’ method in Java. Explain its role in allowing an object to clean up resources before garbage collection. Discuss the importance of proper resource reclamation.
Sample Answer: The ‘finalize’ method in Java allows objects to perform cleanup operations before being garbage collected. It’s crucial for proper resource reclamation, such as releasing file handles or network connections.
Question 54: What Are the Principles of Java Serialization, and How Do You Make a Class Serializable?
How to Respond: Explain your knowledge of Java serialization principles. Describe it as the process of converting objects into byte streams and detail the steps to make a class serializable.
Sample Answer: Java serialization involves converting objects into byte streams. To make a class serializable, it must implement the ‘Serializable’ interface, and its instance variables should be serializable or marked as ‘transient.’
Question 55: Can You Explain the ‘continue’ Statement in Java Loop Control, and Provide Examples of Its Use?
How to Respond: Discuss your understanding of the ‘continue’ statement in Java. Explain that it is used to skip the current iteration in a loop and provide examples to illustrate its usage.
Sample Answer: The ‘continue’ statement in Java is used to skip the current iteration in a loop and proceed with the next. For example, it can be employed to avoid certain loop iterations based on specific conditions.
Question 56: What Is ‘AutoBoxing’ and ‘Unboxing’ in Java, and How Do They Simplify Primitive Data Type Wrapping and Unwrapping?
How to Respond: Describe your knowledge of autoBoxing and unboxing in Java. Explain that autoBoxing is the automatic conversion of primitive data types to wrapper objects, while unboxing is the reverse process. Discuss their benefits.
Sample Answer: AutoBoxing in Java is the automatic conversion of primitive data types to their corresponding wrapper objects. Unboxing is the reverse process. These mechanisms simplify working with primitive data types and wrapper objects, enhancing code readability and convenience.
Question 57: Explain the Purpose of the ‘throws’ Clause in Java Method Signatures and How It Relates to Exception Handling.
How to Respond: Describe the ‘throws’ clause in Java method signatures. Explain its role in declaring exceptions that a method might throw and how it relates to exception handling.
Sample Answer: The ‘throws’ clause in Java method signatures is used to declare exceptions that a method may throw. It provides information to the caller about the potential exceptions that need to be handled.
Question 58: What Is the Difference Between ‘for-each’ Loop and Traditional ‘for’ Loop in Java, and When Would You Use Each?
How to Respond: Compare the ‘for-each’ loop with the traditional ‘for’ loop in Java. Explain their differences and provide scenarios for when to use each loop type.
Sample Answer: In Java, the ‘for-each’ loop is used for iterating through arrays and collections, making code more concise and readable. The traditional ‘for’ loop provides more control over iteration and is suitable for complex loop structures and scenarios requiring precise index control.
Question 59: Can You Explain the Concepts of ‘Aggregation’ and ‘Composition’ in Java, and Provide Examples of Their Use in Class Relationships?
How to Respond: Discuss your knowledge of aggregation and composition in Java class relationships. Explain that aggregation represents a “has-a” relationship, while composition represents a “whole-part” relationship, and provide practical examples.
Sample Answer: In Java, aggregation represents a “has-a” relationship, where one class has another as a part. Composition represents a “whole-part” relationship, where a class is composed of other classes. For instance, a car has an engine (aggregation), and a car consists of wheels, engine, etc. (composition).
Question 60: What Are ‘Static Blocks’ and ‘Static Methods’ in Java Classes, and How Do They Differ from Instance Methods?
How to Respond: Explain your understanding of static blocks and static methods in Java. Describe that static blocks are executed when a class is loaded, while static methods belong to the class rather than instances.
Sample Answer: Static blocks in Java are executed when a class is loaded into memory. Static methods are associated with the class and can be called without creating instances. They differ from instance methods that belong to object instances.
Question 61: What Is ‘Type Casting’ in Java, and How Do You Perform Explicit and Implicit Type Casting? Provide Examples.
How to Respond: Describe ‘type casting’ in Java. Explain that it is the conversion of one data type to another and provide examples of both explicit and implicit type casting.
Sample Answer: Type casting in Java is the conversion of one data type to another. Explicit casting requires a cast operator, e.g., (int) 5.7
, while implicit casting occurs automatically when there’s no data loss, e.g., int num = 5
.
Question 62: Explain the ‘Comparator’ and ‘Comparable’ Interfaces in Java and How They Facilitate Object Comparison and Sorting.
How to Respond: Discuss your knowledge of the ‘Comparator’ and ‘Comparable’ interfaces in Java. Explain their roles in custom object comparison and sorting and provide examples.
Sample Answer: In Java, the ‘Comparator’ interface allows custom object comparison, while the ‘Comparable’ interface is used for natural ordering. Implementing ‘Comparator’ enables flexible sorting, while ‘Comparable’ provides a default sorting order.
Question 63: What Is ‘Synchronization’ in Java Multithreading, and How Does It Ensure Thread Safety in Shared Resources?
How to Respond: Describe ‘synchronization’ in Java multithreading. Explain that it ensures thread safety by allowing only one thread to access a synchronized block or method at a time.
Sample Answer: Synchronization in Java multithreading prevents multiple threads from accessing synchronized code simultaneously. It ensures thread safety in shared resources by allowing only one thread to execute the synchronized block or method at a time.
Question 64: Can You Explain the ‘strictfp’ Keyword in Java, and How Does It Ensure Platform-Independent Floating-Point Calculations?
How to Respond: Discuss your knowledge of the ‘strictfp’ keyword in Java. Explain that it ensures consistent, platform-independent floating-point calculations by restricting optimizations.
Sample Answer: The ‘strictfp’ keyword in Java ensures consistent floating-point calculations across different platforms by restricting optimizations. It guarantees that floating-point operations yield the same results on all systems.
Question 65: What Is ‘Method Chaining’ in Java, and How Is It Achieved? Provide an Example.
How to Respond: Describe ‘method chaining’ in Java. Explain that it involves calling multiple methods on the same object in a single line and provide an example.
Sample Answer: Method chaining in Java allows calling multiple methods on the same object in a single line. For instance, a StringBuilder can be used as follows: StringBuilder sb = new StringBuilder().append("Hello").append(" ").append("World");
Question 66: Explain the Concepts of ‘Polymorphism,’ ‘Inheritance,’ and ‘Encapsulation,’ and How They Contribute to Object-Oriented Programming.
How to Respond: Discuss your understanding of polymorphism, inheritance, and encapsulation in object-oriented programming. Explain their roles in achieving code modularity, reusability, and security.
Sample Answer: In object-oriented programming, polymorphism allows objects to take on multiple forms, inheritance supports code reuse, and encapsulation enhances data security. These principles contribute to modularity, reusability, and maintainability.
Question 67: What Is ‘Reflection’ in Java, and How Can It Be Used to Inspect and Manipulate Classes, Methods, and Fields?
How to Respond: Describe ‘reflection’ in Java. Explain that it enables the inspection and manipulation of class structures, methods, and fields at runtime. Provide examples of reflection usage.
Sample Answer: Reflection in Java allows the dynamic inspection and manipulation of class structures, methods, and fields at runtime. It’s used for tasks like accessing private members, examining annotations, or creating instances of classes.
Question 68: Can You Explain the Purpose and Use of the ‘Enums’ in Java, and How Do They Enhance Code Clarity and Type Safety?
How to Respond: Discuss your knowledge of enums in Java. Explain that they are used to represent a fixed set of named values and how they enhance code clarity and type safety.
Sample Answer: Enums in Java are used to define a fixed set of named values. They enhance code clarity by providing meaningful names for values and ensure type safety by restricting assignments to valid enum constants.
Question 69: What Is ‘Method Overriding’ in Java, and How Does It Support Polymorphism? Provide an Example.
How to Respond: Explain ‘method overriding’ in Java. Describe it as redefining a method in a subclass with the same name, return type, and parameters as the superclass, and provide an example.
Sample Answer: Method overriding in Java involves redefining a method in a subclass with the same name, return type, and parameters as the superclass. It supports polymorphism by allowing different implementations of the same method. For example, in a subclass, you can override a ‘draw’ method to provide a specialized implementation.
Question 70: Can You Describe the Concept of ‘String Pool’ in Java, and How Does It Optimize String Object Creation?
How to Respond: Discuss your understanding of the ‘String Pool’ in Java. Explain that it’s a storage area for string literals, and how it optimizes memory usage and string object creation.
Sample Answer: The ‘String Pool’ in Java is a storage area for string literals. It optimizes memory usage by reusing existing string objects, reducing the creation of duplicate objects for the same string values.
20 Java Basics Interview Questions for Freshers
Question 1: What Is Java, and Why Is It Popular in Software Development?
Question 2: Explain the Key Features of Java as a Programming Language.
Question 3: What Are the Differences Between JDK, JRE, and JVM in Java?
Question 4: How Do You Define a Class and Create an Object in Java?
Question 5: What Is the Main Method, and Why Is It Necessary in Java Programs?
Question 6: What Are Data Types in Java, and How Do You Declare Variables?
Question 7: Explain the Difference Between ‘int’ and ‘Integer’ in Java.
Question 8: What Are Operators in Java, and How Are They Used for Mathematical and Logical Operations?
Question 9: How Do You Perform Input and Output Operations in Java?
Question 10: What Is ‘if-else’ and ‘switch’ Statements in Java, and How Are They Used for Decision Making?
Question 11: Describe the Concepts of ‘while’ and ‘for’ Loops in Java, and Provide Examples of Their Use.
Question 12: What Is an Array in Java, and How Do You Declare and Initialize Arrays?
Question 13: Explain the Importance of ‘try-catch’ Blocks in Handling Exceptions in Java.
Question 14: How Do You Define and Call Methods in Java, and What Are Their Roles in Code Organization?
Question 15: Describe the Concept of ‘Inheritance’ in Java, and How Does It Promote Code Reusability?
Question 16: What Is ‘Polymorphism’ in Java, and How Does It Enable Dynamic Method Invocation?
Question 17: What Are ‘Encapsulation’ and ‘Abstraction,’ and How Do They Enhance Code Security and Clarity?
Question 18: Explain the Purpose of ‘static’ Members in Java Classes and How They Differ from Instance Members.
Question 19: What Are ‘constructors’ in Java, and How Do They Play a Role in Object Initialization?
Question 20: How Do You Manage Memory and Garbage Collection in Java, and Why Is It Important for Program Efficiency?
These questions cover fundamental Java concepts that are often asked in interviews for entry-level positions.
20 Java Basics Interview Questions for Testers
Question 1: What Is Java, and Why Is It Used in Testing and Automation?
Question 2: How Can You Compile and Run a Java Program from the Command Line?
Question 3: Explain the Purpose of the ‘assert’ Statement in Java Testing.
Question 4: What Are JUnit and TestNG, and How Are They Used in Java Testing?
Question 5: How Do You Write and Run a Basic JUnit Test Case in Java?
Question 6: Describe the Different Types of Testing, and How Java Is Used in Each Type.
Question 7: What Are Test Suites in Java Testing, and How Do You Create Them?
Question 8: How Can You Use Annotations to Define Test Methods and Test Execution Order in TestNG?
Question 9: Explain the Role of Mocking Frameworks like Mockito in Java Testing.
Question 10: What Are the Benefits of Parameterized Tests in Java, and How Are They Implemented?
Question 11: How Do You Perform Regression Testing Using Java and Testing Frameworks?
Question 12: Describe the Concept of Code Coverage Analysis and Its Importance in Testing.
Question 13: What Is the Difference Between ‘assertTrue’ and ‘assertEquals’ in JUnit Assertions?
Question 14: How Can You Perform Load Testing and Stress Testing in Java Applications?
Question 15: Explain the ‘WebDriver’ Interface in Selenium and How It Is Used for Web Testing.
Question 16: How Do You Handle Browser Windows and Pop-ups in Selenium Using Java?
Question 17: What Is Page Object Model (POM), and How Does It Enhance Selenium Testing?
Question 18: How Do You Handle Dynamic Elements and Waits in Selenium Java Automation?
Question 19: Explain the Purpose and Use of TestNG Listeners in Java Testing.
Question 20: What Are the Best Practices for Writing and Organizing Test Code in Java Testing Projects?
These questions cover essential Java and testing concepts commonly encountered by testers.
20 Java Basics Interview Questions for Selenium Testers
Question 1: Why Is Java Commonly Used for Selenium Automation Testing?
Question 2: What Are the Key Data Types Used in Selenium Testing with Java?
Question 3: How Do You Initialize WebDriver in Selenium with Java?
Question 4: What Are the Different Types of WebDriver Methods in Selenium?
Question 5: How Do You Handle Web Elements in Selenium Using Java?
Question 6: Explain the Difference Between ‘findElement’ and ‘findElements’ Methods in Selenium.
Question 7: How Do You Perform Basic Navigation and Interactions with Web Pages in Selenium Using Java?
Question 8: What Are Expected Conditions in Selenium, and How Are They Used for Explicit Waits?
Question 9: How Can You Handle Alerts and Pop-ups in Selenium with Java?
Question 10: Explain the Purpose and Usage of Selenium Actions Class in Java.
Question 11: What Is TestNG, and How Does It Facilitate Test Case Management in Selenium Testing with Java?
Question 12: How Do You Execute TestNG Test Suites in Selenium Automation Using Java?
Question 13: What Is Data-Driven Testing in Selenium, and How Can It Be Implemented in Java?
Question 14: How Do You Capture Screenshots in Selenium Test Automation with Java?
Question 15: Describe How to Handle Frames and Windows in Selenium with Java.
Question 16: What Is the Role of Page Object Model (POM) in Selenium Testing, and How Is It Implemented in Java?
Question 17: How Do You Verify Text, Images, and Elements in Selenium Tests Using Java Assertions?
Question 18: What Are the Common Exceptions and How to Handle Them in Selenium Automation with Java?
Question 19: Explain the Importance of Cross-Browser Testing in Selenium, and How Can It Be Achieved with Java?
Question 20: How Do You Generate Test Reports and Logs in Selenium Testing with Java?
These questions cover essential Java-related topics for Selenium testers, helping them navigate automation testing effectively.
Basic Java Interview Questions
- What is Java, and why is it popular in the software development industry?
- Explain the key features of Java as a programming language.
- What are the differences between JDK, JRE, and JVM in Java?
- How do you define a class and create an object in Java?
- What is the main method, and why is it necessary in Java programs?
- Explain the concept of data types in Java and how you declare variables.
- What are operators in Java, and how are they used for mathematical and logical operations?
- How do you perform input and output operations in Java?
- What is conditional branching, and how do ‘if-else’ statements work in Java?
- Describe the purpose and use of loops in Java with examples.
Preparing for a Java Interview
Starting your preparation for a Java interview involves several steps:
- Review Java Basics: Start by revisiting the fundamentals of Java, including syntax, data types, and basic concepts.
- Understand Object-Oriented Programming (OOP): Java is an OOP language, so make sure you are familiar with OOP principles like classes, objects, inheritance, and polymorphism.
- Practice Coding: Solve coding challenges and exercises in Java to improve your problem-solving skills.
- Learn Data Structures and Algorithms: Understand common data structures and algorithms, as they are frequently asked about in technical interviews.
- Study Design Patterns: Familiarize yourself with commonly used design patterns in Java.
- Review Exception Handling: Know how to handle exceptions effectively.
- Practice with Frameworks: If the job requires it, learn about Java frameworks like Spring and Hibernate.
- Prepare for Behavioral Questions: In addition to technical questions, be ready for behavioral questions that assess your teamwork, problem-solving, and communication skills.
Hardest Java Interview Question
The “hardest” Java interview question can vary depending on your level of experience and the specific role you’re applying for. However, some challenging Java interview questions might involve topics like advanced multithreading, memory management, Java performance tuning, or complex algorithmic problems. It’s important to thoroughly understand the basics before tackling these more advanced topics.
Remember that interview difficulty varies from company to company, so it’s a good idea to practice a wide range of Java concepts and problems to be well-prepared.
How do you explain Java in an interview? – a Step by Step Guide
Explaining Java in an interview requires a structured and concise approach. Here’s a step-by-step guide on how to do it effectively:
- Start with a Brief Overview
Begin by giving a high-level overview of Java. You can say something like, “Java is a popular, versatile, and widely-used programming language in the software development industry.”
- Mention Its Key Features
Highlight some key features of Java that set it apart, such as “Java is known for its platform independence, strong support for object-oriented programming, and robustness due to its automatic memory management.”
- Discuss the Role of Java Virtual Machine (JVM)
Explain the importance of JVM in executing Java code. Say, “Java code is compiled into bytecode, which is executed by the Java Virtual Machine (JVM). This bytecode can run on any platform with a compatible JVM, making Java platform-independent.”
- Talk About Object-Oriented Programming (OOP)
Emphasize Java’s strong support for OOP. Mention that “Java encourages the use of classes and objects, making it suitable for building modular and reusable code.”
- Mention Java’s Widespread Usage
Discuss Java’s widespread usage in various domains, such as web development, mobile app development (Android), enterprise applications, and even in embedded systems.
- Highlight Java’s Ecosystem
Mention the rich Java ecosystem, including the Java Standard Library (Java SE) and additional libraries and frameworks for different application types (e.g., Spring for web applications, Hibernate for database access).
- Bring Up Java’s Strong Community and Support
Highlight the active Java community, which provides resources, forums, and support for developers. Mention that Java has a large pool of libraries and resources available.
- Discuss the Portability of Java Code
Explain how Java code can run on different platforms without modification, thanks to the “write once, run anywhere” principle.
- Touch on Java’s Security
Discuss Java’s built-in security features, like the sandboxing of applets and various security measures to protect against vulnerabilities.
- Provide an Example
If possible, provide a simple code snippet or an example that demonstrates the basic structure of a Java program. This can help illustrate your points and make your explanation more concrete.
- Be Prepared for Questions
After your explanation, be ready for follow-up questions. Interviewers may ask about specific Java concepts, its application in real-world projects, or your experience with Java-related tools and frameworks.
- End with Enthusiasm
Conclude your explanation with a positive note, expressing your enthusiasm for Java and your eagerness to apply your knowledge in the job role.
Remember to tailor your explanation to the level of the interview and the specific role you’re applying for. If it’s a technical interview, expect more detailed questions about Java’s inner workings. If it’s a general interview, focus on the broader aspects of Java.
Tips for Cracking Your Java Fundamentals Interview
1. Review the Fundamentals:
- Start by revisiting the core Java concepts, including data types, variables, operators, and basic syntax.
2. Understand Object-Oriented Programming (OOP):
- Java is an object-oriented language, so make sure you grasp OOP principles like classes, objects, inheritance, and polymorphism.
3. Practice Coding:
- Solve coding challenges and exercises in Java to improve your problem-solving skills. Platforms like LeetCode, HackerRank, and CodeSignal offer a variety of Java coding challenges.
4. Master Data Structures and Algorithms:
- Understand common data structures (arrays, lists, maps) and algorithms (sorting, searching) as they are often asked about in technical interviews.
5. Study Java Libraries and APIs:
- Be familiar with Java’s built-in libraries and APIs, such as the Java Collections Framework and the java.util package.
6. Know Exception Handling:
- Learn how to handle exceptions effectively. Be prepared to discuss try-catch blocks and best practices for error handling.
7. Understand the JVM:
- Have a basic understanding of how the Java Virtual Machine (JVM) works, including its role in running Java programs.
8. Brush Up on String Manipulation:
- String manipulation is a common topic in Java interviews. Know how to work with strings, including string concatenation and manipulation methods.
9. Practice with Sample Interview Questions:
- Work through a variety of Java interview questions to become familiar with common patterns and challenges.
10. Study Design Patterns: – Be aware of common design patterns like Singleton, Factory, and Observer. Understand their purposes and when to use them.
11. Review Multithreading: – Basic knowledge of multithreading concepts, such as thread creation and synchronization, is essential, especially for roles involving concurrent programming.
12. Be Ready for Behavioral Questions: – In addition to technical questions, prepare for behavioral questions that assess your teamwork, problem-solving, and communication skills.
13. Ask Questions: – Don’t hesitate to ask for clarification if you don’t understand a question. It’s better to seek clarification than to make assumptions.
14. Stay Calm and Think Aloud: – During the interview, stay composed and express your thought process when solving problems. This helps the interviewer understand your approach.
15. Practice Whiteboard Coding: – If the interview involves whiteboard coding, practice solving problems on a whiteboard or paper to simulate the interview environment.
16. Research the Company: – Before the interview, research the company and its projects. Tailor your responses to show how your skills align with the company’s goals.
17. Mock Interviews: – Consider conducting mock interviews with a friend or mentor to get feedback and improve your interview performance.
18. Stay Up-to-Date: – Keep yourself updated with the latest developments in the Java ecosystem, such as new Java versions and relevant frameworks.
19. Be Enthusiastic: – Show your passion for Java and your eagerness to learn and grow in the field.
Remember, practice and preparation are key. The more you practice and become comfortable with Java concepts, the more confident you’ll feel in your interview. Good luck!