Unlock the power of Java fundamentals with our comprehensive interview question guide. Whether you’re an experienced developer or just starting, this resource is packed with 50+ expertly crafted questions and sample answers to help you shine in your interviews. Our ‘How to Respond Guide’ offers valuable insights, and you can conveniently download it in PDF format. Elevate your Java knowledge and boost your interview success today!
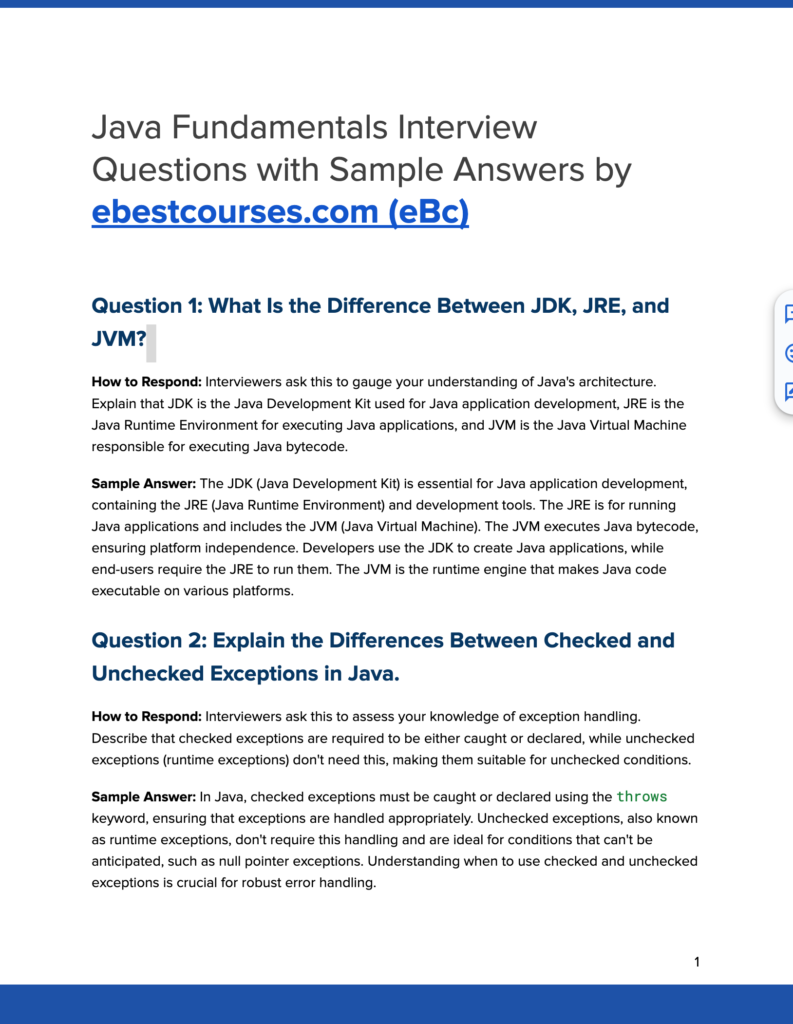
Download Java Fundamentals Interview Questions with Sample Answers PDF
Download our Java Fundamentals Interview Questions PDF for a comprehensive resource featuring questions and expertly crafted sample answers. Prepare to excel in your Java interviews with this valuable reference.
Question 1: What Is the Difference Between JDK, JRE, and JVM?
How to Respond: Interviewers ask this to gauge your understanding of Java’s architecture. Explain that JDK is the Java Development Kit used for Java application development, JRE is the Java Runtime Environment for executing Java applications, and JVM is the Java Virtual Machine responsible for executing Java bytecode.
Sample Answer: The JDK (Java Development Kit) is essential for Java application development, containing the JRE (Java Runtime Environment) and development tools. The JRE is for running Java applications and includes the JVM (Java Virtual Machine). The JVM executes Java bytecode, ensuring platform independence. Developers use the JDK to create Java applications, while end-users require the JRE to run them. The JVM is the runtime engine that makes Java code executable on various platforms.
Question 2: Explain the Differences Between Checked and Unchecked Exceptions in Java.
How to Respond: Interviewers ask this to assess your knowledge of exception handling. Describe that checked exceptions are required to be either caught or declared, while unchecked exceptions (runtime exceptions) don’t need this, making them suitable for unchecked conditions.
Sample Answer: In Java, checked exceptions must be caught or declared using the throws keyword, ensuring that exceptions are handled appropriately. Unchecked exceptions, also known as runtime exceptions, don’t require this handling and are ideal for conditions that can’t be anticipated, such as null pointer exceptions. Understanding when to use checked and unchecked exceptions is crucial for robust error handling.
Question 3: What Is the Role of the final
Keyword in Java?
How to Respond: Interviewers ask this to test your knowledge of the final
keyword. Explain that final
is used to declare constants, make variables unchangeable, or prevent method overriding in subclasses.
Sample Answer: In Java, the final keyword serves various purposes. It’s used to declare constants, creating values that can’t be modified. It also prevents variables from being reassigned after initialization. Additionally, it’s used to mark methods, preventing them from being overridden in subclasses. The final keyword ensures immutability and enhances code security and performance.
Question 4: Describe the Differences Between ArrayList
and LinkedList
in Java.
How to Respond: Interviewers ask this to evaluate your knowledge of different collection types. Explain that ArrayList
is backed by an array and suitable for random access, while LinkedList
uses a doubly-linked list and is efficient for adding/removing elements.
Sample Answer: In Java, ArrayList and LinkedList are both list implementations. ArrayList is backed by an array, which provides fast random access but can be slow for insertions and deletions. On the other hand, LinkedList uses a doubly-linked list, making it efficient for adding or removing elements, especially in the middle of the list. Choosing between them depends on the specific requirements of your application.
Question 5: What Is the Purpose of the equals()
and hashCode()
Methods in Java?
How to Respond: Interviewers ask this to assess your knowledge of object comparison and hashing. Explain that equals()
is used to compare object content, while hashCode()
generates a unique code for objects stored in collections like HashMap
.
Sample Answer: In Java, the equals() method is used for comparing the content of objects, typically overridden in custom classes to define equality criteria. The hashCode() method generates a unique hash code for objects, often used for efficient storage and retrieval in collections like HashMap. Implementing these methods correctly is vital for object comparison and hashing.
Question 6: What Is the Purpose of the static
Keyword in Java?
How to Respond: Interviewers ask this to evaluate your understanding of static members. Explain that static
is used to create class-level members shared by all instances, such as variables and methods.
Sample Answer: The static keyword in Java is used to create class-level members, which are shared by all instances of a class. Static variables and methods belong to the class rather than individual objects, making them accessible without object instantiation. Common use cases include defining constants, counting instances, and providing utility methods. Understanding when to use static members is crucial for efficient and organized code.
Question 7: Explain the Concepts of Method Overloading and Method Overriding in Java.
How to Respond: Interviewers ask this to test your knowledge of method behavior. Describe that method overloading allows multiple methods with the same name but different parameters, while method overriding is the replacement of a superclass method in a subclass with a specialized implementation.
Sample Answer: Method overloading in Java enables the existence of multiple methods with the same name but different parameters within a class. This provides flexibility in method calling. On the other hand, method overriding occurs in inheritance when a subclass replaces a method in the superclass with its specific implementation. Overriding is essential for achieving polymorphism and tailoring methods to suit specific object types.
Question 8: What Is the Java Memory Management System, and How Does Garbage Collection Work?
How to Respond: Interviewers ask this to gauge your knowledge of memory management. Explain that Java uses automatic memory management through garbage collection, where the JVM reclaims memory from objects no longer in use.
Sample Answer: In Java, the memory management system relies on automatic garbage collection. The JVM continuously monitors memory allocation and reclaims memory from objects that are no longer accessible, preventing memory leaks. It uses various algorithms to identify and remove unreferenced objects. Garbage collection is a fundamental Java feature that ensures efficient memory usage and eliminates manual memory management, making Java robust and secure.
Question 9: What Are Constructors in Java, and Why Are They Important?
How to Respond: Interviewers ask this to assess your understanding of constructors. Explain that constructors are special methods used to initialize objects and play a crucial role in object creation.
Sample Answer: Constructors in Java are special methods used for initializing objects when they are created. They have the same name as the class and no return type. Constructors play a vital role in the object-oriented paradigm, ensuring that objects start with valid states. They allow you to set initial values, perform necessary setups, and ensure that objects are ready for use. Understanding constructors is fundamental in Java programming.
Question 10: What Is the Difference Between ==
and .equals()
for Comparing Objects in Java?
How to Respond: Interviewers ask this to evaluate your knowledge of object comparison. Explain that ==
compares object references, while .equals()
is used to compare object content.
Sample Answer: In Java, == compares object references to determine if two references point to the same object in memory. On the other hand, .equals() is a method used to compare the content of objects. It can be overridden in custom classes to define equality criteria based on the object’s state. Understanding when to use == for reference comparison and .equals() for content comparison is crucial for accurate object comparisons.
Question 11: What Is a Java Interface, and How Does It Differ from a Class?
How to Respond: Interviewers ask this to assess your understanding of interfaces. Explain that a Java interface defines a contract for classes but cannot be instantiated. Differentiate it from classes and clarify the use of multiple interfaces.
Sample Answer: In Java, an interface is a blueprint for classes to follow, defining a set of abstract methods. It cannot be instantiated like a class. Unlike classes, which can have fields and concrete methods, interfaces only contain method signatures. A class can implement multiple interfaces, enabling it to adhere to multiple contracts. Interfaces are essential for achieving abstraction and providing a clear separation between contracts and implementations.
Question 12: What Is the NullPointerException
in Java, and How Can It Be Prevented?
How to Respond: Interviewers ask this to evaluate your error-handling knowledge. Explain that NullPointerException
is a runtime exception when trying to access a null object. Discuss prevention strategies, such as null checks.
Sample Answer: In Java, a NullPointerException occurs when attempting to access or manipulate an object that is null. To prevent it, always check for null before accessing objects, use conditional statements, or employ the null-safe operator (Java 14+). Additionally, design your code with proper initialization practices to ensure that variables and objects are initialized before use. Handling nulls effectively is essential for robust and reliable Java applications.
Question 13: What Are the Differences Between for
and while
Loops in Java? Provide Code Examples.
How to Respond: Interviewers ask this to assess your knowledge of loop constructs. Describe that both loops control the flow of execution but differ in syntax and use cases.
Sample Answer: In Java, for and while loops both control program flow, but they differ in syntax and use cases. A for loop is ideal for iterating a known number of times, with its syntax explicitly stating initialization, condition, and iteration. A while loop is more flexible and suitable for indefinite iterations based on a condition. Here are examples:
// Using a for loop
for (int i = 0; i < 5; i++) {
// Code to execute
}
// Using a while loop
int count = 0;
while (count < 5) {
// Code to execute
count++;
}
Question 14: What Is Polymorphism in Java, and How Is It Achieved?
How to Respond: Interviewers ask this to test your understanding of polymorphism. Explain that polymorphism enables objects to be treated as instances of their parent class or interfaces, achieved through method overriding and inheritance.
Sample Answer: In Java, polymorphism allows objects to be treated as instances of their parent class or implemented interfaces. It is achieved through method overriding, where a subclass provides a specific implementation of a method defined in its superclass. Polymorphism is fundamental in achieving code flexibility and reusability, as it allows multiple objects to respond to a method call differently based on their specific implementations.
Question 15: What Is the this
Keyword in Java, and How Is It Used? Provide Code Examples.
How to Respond: Interviewers ask this to assess your understanding of the this
keyword. Explain that this
refers to the current object instance and is used to differentiate instance variables from method parameters.
Sample Answer: In Java, the this keyword refers to the current object instance. It is commonly used to disambiguate between instance variables and method parameters when they share the same name. Here's an example:
public class MyClass {
private int value;
public void setValue(int value) {
this.value = value; // 'this' distinguishes the instance variable from the parameter
}
}
Question 16: What Is Inheritance in Java, and How Does It Facilitate Code Reuse?
How to Respond: Interviewers ask this to assess your understanding of inheritance. Explain that inheritance allows a subclass to inherit properties and behaviors from a superclass, promoting code reuse and extensibility.
Sample Answer: In Java, inheritance is a fundamental concept that enables a subclass to inherit properties and behaviors from a superclass. It promotes code reuse and extensibility, as a subclass can leverage the attributes and methods of its superclass. Inheritance establishes an "is-a" relationship, where a subclass is a specific type of its superclass. By inheriting from a superclass, a subclass can enhance, modify, or extend the inherited functionality, leading to a structured and efficient codebase.
Question 17: What Is the Purpose of the super
Keyword in Java, and How Is It Used in Inheritance?
How to Respond: Interviewers ask this to evaluate your knowledge of the super
keyword. Explain that super
is used to access and call methods or constructors from the superclass and is especially helpful when dealing with method overriding.
Sample Answer: In Java, the super keyword is used to access methods or constructors from the superclass. It is particularly valuable in cases of method overriding, allowing the subclass to call the overridden method of the superclass. The super keyword provides a way to extend or customize the behavior of a superclass method while retaining the original functionality. It is crucial for maintaining the integrity of the inheritance hierarchy.
Question 18: What Are Java Annotations, and How Are They Used in Code? Provide Examples.
How to Respond: Interviewers ask this to assess your understanding of Java annotations. Explain that annotations provide metadata about code elements and are used for various purposes like documentation, code analysis, and frameworks.
Sample Answer: In Java, annotations are a form of metadata that can be added to code elements like classes, methods, and fields. They provide information about code and are used for documentation, code analysis, and frameworks. Examples include @Override, used to indicate method overriding, and custom annotations like @Deprecated or @Test used for various purposes. Annotations are essential for enhancing code clarity and providing additional information to tools and frameworks.
Question 19: What Is a Java Enum, and How Are Enumerations Used in Code?
How to Respond: Interviewers ask this to evaluate your knowledge of enumerations. Explain that a Java enum is a special data type for defining a set of constants and how they can be used for representing fixed values.
Sample Answer: In Java, an enum is a data type used to define a set of constants. Enums are often used to represent fixed values like days of the week, months, or status codes. They ensure that variables can only be assigned one of the predefined values, enhancing code readability and reducing errors. Here's an example of a simple enum:
enum Day {
SUNDAY, MONDAY, TUESDAY, WEDNESDAY, THURSDAY, FRIDAY, SATURDAY
}
Question 20: What Is Exception Chaining in Java, and Why Is It Useful? Provide an Example.
How to Respond: Interviewers ask this to assess your knowledge of exception handling. Explain that exception chaining involves wrapping one exception inside another and why it's beneficial for preserving the context of the original exception.
Sample Answer: Exception chaining in Java involves wrapping one exception inside another, creating a chain of exceptions. This is particularly useful for preserving the context of the original exception while adding additional information or context. It allows developers to understand the cause of an exception more clearly. Here's an example of exception chaining:
try {
// Code that may throw an exception
} catch (IOException e) {
throw new MyCustomException("An error occurred", e);
}
Question 21: What Is the transient
Keyword in Java, and When Is It Used in Serialization?
How to Respond: Interviewers ask this to evaluate your knowledge of object serialization. Explain that the transient
keyword is used to indicate that a field should not be serialized and is valuable for excluding sensitive or non-serializable data.
Sample Answer: In Java, the transient keyword is used to indicate that a field should not be serialized when an object is converted to a stream, such as for storage or network transmission. This is valuable when you have fields that contain sensitive data, or data that doesn't make sense to be serialized, like open network connections or GUI components. Marking a field as transient ensures it is excluded from the serialization process.
Question 22: What Is the Purpose of the switch
Statement in Java, and How Does It Differ from if-else
Statements?
How to Respond: Interviewers ask this to evaluate your knowledge of control flow constructs. Explain that the switch
statement is used for multi-branch decision-making based on a single expression, while if-else
statements allow more complex conditions.
Sample Answer: In Java, the switch statement is used for multi-branch decision-making based on a single expression. It simplifies code when multiple conditions depend on the same variable's value. On the other hand, if-else statements are more versatile and allow for more complex conditions with multiple expressions. Use a switch when you have straightforward, value-based branching, and if-else for more intricate conditions.
Question 23: How Do You Create and Use Java Annotations in Your Code? Provide an Example.
How to Respond: Interviewers ask this to assess your knowledge of custom annotations. Explain how to create and use custom annotations in code, and provide an example of their application.
Sample Answer: In Java, you can create custom annotations by defining them with the @interface keyword. These annotations can then be applied to code elements like classes, methods, or fields to provide additional metadata. Here's an example of a simple custom annotation:
@interface MyAnnotation {
String value();
}
@MyAnnotation("This is an example annotation")
public class MyClass {
// Code here
}
Custom annotations are used for documentation, code analysis, and creating custom behaviors or frameworks.
Question 24: What Is the Purpose of the volatile
Keyword in Java, and How Does It Affect Variables?
How to Respond: Interviewers ask this to evaluate your knowledge of multithreading. Explain that volatile
is used to mark a variable as "volatile," indicating that its value may be modified by multiple threads and should be synchronized accordingly.
Sample Answer: In Java, the volatile keyword is used to mark a variable as "volatile," indicating that its value may be modified by multiple threads. This ensures that any read or write operation on a volatile variable is atomic and synchronized. It is used when variables are accessed by multiple threads and must maintain a consistent, shared state. The volatile keyword helps prevent thread-related issues like data races and ensures safe concurrent access to the variable.
Question 25: How Does Java Support Multithreading, and What Is the Role of the Thread
Class?
How to Respond: Interviewers ask this to assess your understanding of multithreading in Java. Explain that Java provides multithreading support through the Thread
class and the Runnable
interface.
Sample Answer: Java supports multithreading by providing the Thread class and the Runnable interface. The Thread class is used to create and manage threads, while the Runnable interface defines the entry point for thread execution. Developers can extend the Thread class or implement the Runnable interface to create and execute threads. Multithreading enables concurrent execution, allowing applications to perform tasks in parallel, enhancing performance, and responsiveness.
Question 26: What Is the Difference Between the StringBuilder
and StringBuffer
Classes in Java?
How to Respond: Interviewers ask this to assess your knowledge of string manipulation. Explain that both classes provide mutable strings, but StringBuilder
is not synchronized, making it more efficient, while StringBuffer
is synchronized but slower.
Sample Answer: In Java, both StringBuilder and StringBuffer provide mutable strings, allowing efficient string manipulation. The key difference is that StringBuilder is not synchronized, making it faster for single-threaded operations, while StringBuffer is synchronized, ensuring thread safety but resulting in slightly slower performance. Developers choose between them based on their specific requirements, favoring StringBuilder for most non-concurrent scenarios due to its efficiency.
Question 27: What Are Java Annotations, and How Are They Used in Code? Provide Examples of Built-in Annotations.
How to Respond: Interviewers ask this to evaluate your knowledge of built-in annotations. Explain that annotations are metadata added to code elements and provide examples of built-in annotations like @Override
and @Deprecated
.
Sample Answer: In Java, annotations are metadata added to code elements to provide additional information. Built-in annotations include @Override (used to indicate method overriding) and @Deprecated (signaling that a method or class is outdated and should not be used). Here are examples:
@Override
public void myMethod() {
// Method implementation
}
@Deprecated
public class OldClass {
// Code here
}
Annotations enhance code clarity, documentation, and can be used by tools and frameworks to enforce specific behaviors.
Question 28: What Is Java Reflection, and How Can It Be Used in Code? Provide an Example.
How to Respond: Interviewers ask this to assess your knowledge of reflection. Explain that Java Reflection allows you to inspect and manipulate classes, methods, fields, and objects at runtime, and provide an example.
Sample Answer: Java Reflection is a powerful feature that enables the inspection and manipulation of classes, methods, fields, and objects at runtime. It allows you to obtain class information, access fields, and invoke methods dynamically. Here's a simple example of using reflection to invoke a method:
Class> myClass = MyClass.class;
Method myMethod = myClass.getMethod("myMethod", parameterTypes);
Object instance = myClass.getDeclaredConstructor().newInstance();
Object result = myMethod.invoke(instance, arguments);
Reflection is especially useful for scenarios like dynamic class loading and working with unknown or external classes.
Question 29: What Is Java Serialization, and How Does It Work? Provide an Example of Serializing and Deserializing Objects.
How to Respond: Interviewers ask this to assess your knowledge of object serialization. Explain that Java serialization is the process of converting objects into a byte stream and vice versa, and provide an example of serializing and deserializing objects.
Sample Answer: Java serialization is the process of converting objects into a byte stream for storage or transmission, and deserialization is the reverse process of recreating objects from the byte stream. Here's an example:
// Serialize an object
ObjectOutputStream out = new ObjectOutputStream(new FileOutputStream("data.ser"));
out.writeObject(myObject);
out.close();
// Deserialize an object
ObjectInputStream in = new ObjectInputStream(new FileInputStream("data.ser"));
MyClass myObject = (MyClass) in.readObject();
in.close();
Serialization is used for data persistence, network communication, and more.
Question 30: What Is the Purpose of the try-catch-finally
Block in Java Exception Handling? Provide an Example.
How to Respond: Interviewers ask this to assess your knowledge of exception handling. Explain that the try-catch-finally
block is used to handle exceptions, and provide an example.
Sample Answer: In Java, the try-catch-finally block is used for exception handling. The try block encloses code that might throw exceptions, the catch block handles specific exception types, and the finally block ensures that certain code executes regardless of whether an exception occurs. Here's an example:
try {
// Code that may throw an exception
} catch (MyException e) {
// Handle MyException
} finally {
// Cleanup or resource release
}
The try-catch-finally block is vital for maintaining program stability and managing resources, ensuring graceful error handling.
Question 31: What Are Lambda Expressions in Java, and How Are They Used? Provide an Example.
How to Respond: Interviewers ask this to evaluate your knowledge of functional programming in Java. Explain that lambda expressions are anonymous functions used for concise code and provide an example.
Sample Answer: Lambda expressions in Java are anonymous functions that allow you to write compact and more readable code. They are often used for functional programming and can be used to define inline implementations of functional interfaces. Here's an example of a lambda expression for a Runnable:
Runnable myRunnable = () -> {
System.out.println("This is a lambda expression.");
};
Lambda expressions simplify code, making it more expressive and efficient when working with functional interfaces.
Question 32: What Is Java Generics, and How Do They Improve Type Safety in Code?
How to Respond: Interviewers ask this to assess your knowledge of generics. Explain that generics enable type-safe code by allowing you to specify the data type of objects used in collections, classes, and methods.
Sample Answer: Java generics provide a way to create classes, interfaces, and methods that operate on specified types. They improve type safety by allowing you to define the data type of objects used in collections, classes, and methods. This prevents runtime type errors, making your code more reliable and readable. Generics are extensively used in Java libraries for container classes like ArrayList
Question 33: What Is the Purpose of the default
Method in Java Interfaces, and How Is It Used? Provide an Example.
How to Respond: Interviewers ask this to evaluate your knowledge of default methods in interfaces. Explain that default
methods provide default implementations in interfaces and provide an example.
Sample Answer: In Java, default methods in interfaces allow you to provide default implementations for interface methods. This is useful when adding new methods to existing interfaces without breaking classes that implement them. Here's an example:
public interface MyInterface {
void myMethod();
default void myDefaultMethod() {
System.out.println("This is a default method.");
}
}
Default methods enhance interface extensibility without affecting implementing classes.
Question 34: What Are the Differences Between the String
and StringBuilder
Classes in Java?
How to Respond: Interviewers ask this to assess your knowledge of string manipulation. Explain that String
is immutable, while StringBuilder
is mutable, making it more efficient for concatenating and modifying strings.
Sample Answer: In Java, the String class is immutable, meaning once a String object is created, its value cannot be changed. In contrast, the StringBuilder class is mutable, allowing for efficient string concatenation and modification. When you frequently modify or build strings, using StringBuilder is more efficient because it avoids the overhead of creating new String objects.
Question 35: What Is the AutoCloseable
Interface in Java, and How Does It Improve Resource Management? Provide an Example.
How to Respond: Interviewers ask this to assess your knowledge of resource management. Explain that the AutoCloseable
interface is used for automatic resource management, and provide an example.
Sample Answer: In Java, the AutoCloseable interface is used for automatic resource management. It provides a way to ensure that resources like file handles or database connections are properly closed when they are no longer needed. Here's an example:
try (MyResource resource = new MyResource()) {
// Use the resource
} catch (Exception e) {
// Handle exceptions
}
The try-with-resources statement automatically closes the resource when the block is exited, enhancing code reliability and resource management.
Question 36: What Is Method Reference in Java, and How Does It Simplify Code? Provide Examples of Method References.
How to Respond: Interviewers ask this to assess your knowledge of method references. Explain that method reference is a shorthand notation for invoking methods and provide examples.
Sample Answer: In Java, method reference is a shorthand notation for invoking methods. It simplifies code and can be used with functional interfaces. There are different types of method references, such as referencing a static method or an instance method of an object. Here are examples:
// Reference to a static method
Function parser = Integer::parseInt;
// Reference to an instance method of an object
List list = new ArrayList<>();
Consumer consumer = list::add;
Method references make code more concise and expressive, especially when working with functional interfaces.
Question 37: What Is Java NIO, and How Does It Improve I/O Performance?
How to Respond: Interviewers ask this to assess your knowledge of Java NIO (New I/O). Explain that Java NIO is a framework for high-performance, non-blocking I/O operations and how it improves I/O performance.
Sample Answer: Java NIO (New I/O) is a framework that provides high-performance, non-blocking I/O operations. It improves I/O performance by offering features like channels and buffers, making it suitable for scalable and responsive applications. NIO is especially valuable when working with large data sets or concurrent connections, as it avoids the overhead of traditional blocking I/O, enhancing application efficiency and responsiveness.
Question 38: What Is Java EnumSet, and How Is It Used in Code? Provide an Example.
How to Respond: Interviewers ask this to assess your knowledge of Java EnumSet. Explain that EnumSet is a specialized set implementation for enums, and provide an example.
Sample Answer:
javaCopy code
enum Day { SUNDAY, MONDAY, TUESDAY, WEDNESDAY, THURSDAY, FRIDAY, SATURDAY } // Create an EnumSet EnumSet<Day> weekend = EnumSet.of(Day.SUNDAY, Day.SATURDAY);
Sample Answer: In Java, an EnumSet is a specialized set implementation designed for use with enum types. It provides efficient and type-safe storage for enum values. Here's an example:
enum Day { SUNDAY, MONDAY, TUESDAY, WEDNESDAY, THURSDAY, FRIDAY, SATURDAY }
// Create an EnumSet
EnumSet weekend = EnumSet.of(Day.SUNDAY, Day.SATURDAY);
EnumSet is used when you need to work with enum values in a set-like structure, ensuring type safety and performance.
Question 39: What Is the Purpose of Java Reflection, and How Is It Used to Inspect and Modify Code at Runtime?
How to Respond: Interviewers ask this to assess your knowledge of reflection. Explain that Java Reflection allows you to inspect and modify code at runtime, and provide examples.
Sample Answer:
javaCopy code
Class<?> myClass = MyClass.class; Field myField = myClass.getDeclaredField("myPrivateField"); myField.setAccessible(true); Object value = myField.get(myInstance);
Sample Answer: Java Reflection is a feature that enables the inspection and modification of code at runtime. It allows you to access class information, fields, methods, and objects dynamically. Reflection is used for various purposes, such as creating plugins, working with unknown classes, or implementing advanced debugging tools. Here's an example of using reflection to access a private field:
Class> myClass = MyClass.class;
Field myField = myClass.getDeclaredField("myPrivateField");
myField.setAccessible(true);
Object value = myField.get(myInstance);
Reflection provides powerful capabilities for dynamic code manipulation, but it should be used judiciously due to its potential complexity and security implications.
Question 40: What Are Checked and Unchecked Exceptions in Java, and How Should They Be Handled Differently?
How to Respond: Interviewers ask this to assess your knowledge of exception handling. Explain the difference between checked and unchecked exceptions and how they should be handled.
Sample Answer: In Java, exceptions are categorized into checked and unchecked exceptions. Checked exceptions are those that must be either caught or declared in a method's signature, ensuring that the exception is handled by the caller. Unchecked exceptions (also known as runtime exceptions) do not need to be declared or caught explicitly.
Checked exceptions are typically used for recoverable errors, like file not found, where the application can take corrective action. Unchecked exceptions are often related to programming errors, like null pointer exceptions, and are generally not recoverable.
Handling checked and unchecked exceptions differs in how they are caught and treated. Checked exceptions should be explicitly caught, and recovery or error-handling mechanisms should be implemented. Unchecked exceptions are typically a result of programming errors and should be addressed by improving the code to prevent them.
These questions cover a range of Java topics, from advanced concepts like reflection and NIO to essential elements like exceptions and method references. If you have any specific areas you'd like to explore further or have more questions in mind, please let me know.
Question 41: What Is Java Stream API, and How Does It Simplify Data Processing? Provide an Example.
How to Respond: Interviewers ask this to assess your knowledge of Java Stream API. Explain that the Stream API simplifies data processing by providing functional-style operations on collections, and provide an example.
Sample Answer: Java Stream API simplifies data processing by offering functional-style operations on collections. It allows developers to perform complex transformations, filtering, and computations with concise, readable code. Here's an example of using streams to find the sum of even numbers in a list:
List numbers = Arrays.asList(1, 2, 3, 4, 5, 6, 7, 8, 9, 10);
int sum = numbers.stream()
.filter(n -> n % 2 == 0)
.mapToInt(Integer::intValue)
.sum();
Stream API enhances code readability and maintainability when working with data.
Question 42: What Are Java Annotations, and How Are They Used in Frameworks Like Spring? Provide Examples.
How to Respond: Interviewers ask this to evaluate your knowledge of annotations in frameworks. Explain that annotations are used for configuration and customization in frameworks like Spring and provide examples.
Sample Answer: In Java, annotations are used for providing metadata about code elements. In frameworks like Spring, annotations play a crucial role in configuration and customization. Examples include @Component for marking a class as a Spring component and @Autowired for injecting dependencies. Here's an example:
@Component
public class MyService {
// Code here
}
@Service
public class MyOtherService {
@Autowired
private MyService service;
}
Annotations simplify configuration and allow developers to focus on application logic.
Question 43: What Are the Benefits of Using Java 8's java.time
API for Date and Time Handling? Provide Examples.
How to Respond: Interviewers ask this to assess your knowledge of the java.time
API. Explain that it provides improved date and time handling with immutability, better readability, and time zone support, and provide examples.
Sample Answer: Java 8 introduced the java.time API, offering several benefits for date and time handling. It provides immutability, enhanced readability, and comprehensive support for time zones. Here's an example of parsing and formatting a date:
// Parse a date string
LocalDate date = LocalDate.parse("2023-10-28");
// Format a date
DateTimeFormatter formatter = DateTimeFormatter.ofPattern("MMM d, yyyy");
String formattedDate = date.format(formatter);
The java.time API simplifies date and time operations and helps avoid common date-related bugs.
Question 44: What Is Java RMI, and How Does It Facilitate Remote Method Invocation? Provide an Example.
How to Respond: Interviewers ask this to assess your knowledge of Java RMI (Remote Method Invocation). Explain that RMI enables remote communication between objects in distributed systems, and provide an example.
Sample Answer: Java RMI (Remote Method Invocation) facilitates remote communication between objects in distributed systems. It allows objects to invoke methods on objects running in different Java virtual machines. Here's a simplified example:
// Define a remote interface
public interface MyRemoteInterface extends Remote {
String sayHello() throws RemoteException;
}
// Implement the interface
public class MyRemoteObject extends UnicastRemoteObject implements MyRemoteInterface {
public MyRemoteObject() throws RemoteException {
}
@Override
public String sayHello() throws RemoteException {
return "Hello, remote world!";
}
}
// Create and export the remote object
MyRemoteInterface remoteObject = new MyRemoteObject();
Registry registry = LocateRegistry.getRegistry();
registry.bind("MyRemoteObject", remoteObject);
Java RMI enables remote method invocation, making it suitable for distributed applications.
Question 45: What Is the Purpose of Java Optional
and How Does It Improve Null Safety? Provide Examples.
How to Respond: Interviewers ask this to assess your knowledge of Optional
in Java. Explain that Optional
is used for null safety, and provide examples.
Sample Answer: Java's Optional is a class used to address null safety issues by representing a value that may or may not be present. It encourages proper handling of potentially absent values. Here's an example:
Optional maybeName = Optional.ofNullable(getName());
String result = maybeName.orElse("Unknown");
// With Java 9+
String result = maybeName.orElseGet(() -> "Unknown");
Optional helps avoid null references and provides a clear way to handle cases where a value might be missing.
Question 46: What Is the Purpose of Java Annotations, and How Are They Used in JUnit Testing? Provide Examples.
How to Respond: Interviewers ask this to evaluate your knowledge of annotations in JUnit testing. Explain that annotations are used for test configuration and execution in JUnit, and provide examples.
Sample Answer: Java annotations are used in JUnit testing to configure and execute test cases. Examples include @Test for marking test methods and @Before and @After for setup and teardown methods. Here's an example:
public class MyTest {
@Before
public void setUp() {
// Initialize test environment
}
@Test
public void testSomething() {
// Test logic
}
@After
public void tearDown() {
// Cleanup after the test
}
}
Annotations in JUnit simplify test case management and execution.
Question 47: What Is Java Collections Framework, and How Does It Enhance Data Structure Management?
How to Respond: Interviewers ask this to assess your knowledge of the Java Collections Framework. Explain that it provides a unified set of interfaces and classes for managing data structures, and how it simplifies data manipulation.
Sample Answer: The Java Collections Framework is a comprehensive set of interfaces and classes for managing and manipulating data structures like lists, sets, and maps. It provides a unified and consistent way to work with collections, enhancing code reusability and readability. With the framework, developers can choose the most suitable data structure for their specific needs, making data manipulation more efficient and error-resistant.
Question 48: What Are Java Applets, and How Are They Used for Web-Based Applications?
How to Respond: Interviewers ask this to assess your knowledge of Java applets. Explain that applets are small Java programs designed to run within web browsers, and how they are used in web-based applications.
Sample Answer: Java applets are small Java programs designed to run within web browsers. They were historically used for interactive and dynamic web-based applications. Applets could be embedded in web pages and provide a richer user experience than standard HTML. However, their usage has significantly declined in favor of other web technologies like JavaScript and HTML5 due to security concerns and compatibility issues with modern browsers.
Question 49: What Is the Java ClassLoader, and How Does It Load Classes at Runtime? Provide Examples.
How to Respond: Interviewers ask this to assess your knowledge of class loading in Java. Explain that the Java ClassLoader loads classes at runtime, and provide examples.
Sample Answer: In Java, the ClassLoader is responsible for loading classes at runtime. It uses a delegation hierarchy to search for classes in different locations, such as the system classpath or custom class loaders. Here's a simple example of a custom class loader:
class MyClassLoader extends ClassLoader {
public Class> findClass(String className) {
// Load the class from a custom source
}
}
MyClassLoader classLoader = new MyClassLoader();
Class> myClass = classLoader.loadClass("com.example.MyClass");
Class loading is a fundamental concept for dynamic loading and unloading of classes during runtime.
Question 50: What Are the Key Principles of Object-Oriented Programming (OOP) in Java, and How Do They Enhance Software Design?
How to Respond: Interviewers ask this to assess your understanding of OOP principles. Explain key principles like encapsulation, inheritance, polymorphism, and abstraction, and how they improve software design.
Sample Answer: Object-Oriented Programming (OOP) in Java is based on several key principles. Encapsulation ensures that data is hidden within objects and can only be accessed through well-defined interfaces, enhancing data security and code maintainability. Inheritance allows classes to inherit properties and behaviors from other classes, promoting code reuse and hierarchy. Polymorphism enables objects to take on multiple forms, making code more flexible and extensible. Abstraction simplifies complex systems by focusing on relevant details while hiding unnecessary complexity.
Question 51: What Is Java Bytecode, and How Does It Facilitate Platform Independence?
How to Respond: Interviewers ask this to assess your understanding of Java bytecode. Explain that bytecode is an intermediate representation of Java source code and how it enables platform independence.
Sample Answer: Java bytecode is an intermediate representation of Java source code that is generated during the compilation process. It is not machine-specific, which makes Java platform-independent. When a Java program is compiled, it is transformed into bytecode, which can then be executed on any platform that has a Java Virtual Machine (JVM) implementation. This allows developers to write code once and run it anywhere, a key feature of Java's "Write Once, Run Anywhere" philosophy.
Question 52: What Are Java Annotations, and How Are They Used in Spring Framework for Dependency Injection? Provide Examples.
How to Respond: Interviewers ask this to assess your knowledge of annotations in the Spring framework. Explain that annotations are used for configuring dependency injection and provide examples.
Sample Answer: In the Spring framework, annotations play a vital role in configuring and managing dependency injection. Examples include @Component to mark a class as a Spring component, @Autowired to inject dependencies, and @Qualifier for specifying the exact bean to inject. Here's an example:
@Component
public class MyService {
// Code here
}
@Service
public class MyOtherService {
@Autowired
@Qualifier("myService")
private MyService service;
}
Annotations in Spring simplify configuration and enhance code readability, promoting a more efficient development process.
Question 53: What Is the Purpose of the volatile
Keyword in Java, and How Does It Ensure Thread Safety? Provide Examples.
How to Respond: Interviewers ask this to assess your knowledge of thread safety. Explain that the volatile
keyword is used to guarantee visibility of a variable's changes among threads and provide examples.
Sample Answer: In Java, the volatile keyword is used to ensure that changes to a variable are immediately visible to other threads. It prevents thread-local caching of the variable, promoting consistent and reliable communication between threads. Here's an example:
class MySharedResource {
private volatile boolean flag = false;
public void setFlagTrue() {
flag = true;
}
public boolean isFlag() {
return flag;
}
}
Using volatile is crucial in scenarios where multiple threads access the same variable to maintain thread safety and avoid race conditions.
Question 54: What Is a Java Servlet, and How Is It Used for Developing Web Applications? Provide Examples.
How to Respond: Interviewers ask this to assess your knowledge of Java Servlets. Explain that Servlets are Java components used for building web applications, and provide examples.
Sample Answer: Java Servlets are Java components that extend the functionality of a web server to provide dynamic and interactive web applications. They receive and respond to client requests, typically over HTTP. Here's a simple example of a basic servlet:
import javax.servlet.*;
import java.io.IOException;
public class MyServlet implements Servlet {
public void init(ServletConfig config) throws ServletException {
// Initialization code
}
public void service(ServletRequest request, ServletResponse response) throws ServletException, IOException {
// Processing of client request and generating response
}
public void destroy() {
// Cleanup code
}
public ServletConfig getServletConfig() {
return null;
}
public String getServletInfo() {
return null;
}
}
Servlets are the foundation for Java web applications and can handle various aspects of web development, such as processing forms, interacting with databases, and generating dynamic content.
Question 55: What Are Inner Classes in Java, and How Are They Used for Encapsulation? Provide Examples.
How to Respond: Interviewers ask this to evaluate your knowledge of inner classes. Explain that inner classes are classes within other classes, used for encapsulation, and provide examples.
Sample Answer: In Java, inner classes are classes defined within other classes. They are used to achieve encapsulation by keeping related classes close together and hidden from outside access. Here's an example of an inner class:
public class Outer {
private int outerField;
class Inner {
private int innerField;
public void doSomething() {
// Access outer and inner fields
}
}
}
Inner classes can access the fields and methods of their enclosing class, making them suitable for implementing design patterns, like the Iterator pattern, and improving code organization.
Question 56: What Is the Purpose of the transient
Keyword in Java, and How Is It Used in Serialization? Provide Examples.
How to Respond: Interviewers ask this to assess your knowledge of Java object serialization. Explain that the transient
keyword is used to exclude fields from serialization, and provide examples.
Sample Answer: In Java, the transient keyword is used to exclude specific fields from the serialization process. This is useful when certain fields should not be included in the serialized object. Here's an example:
class MySerializableObject implements Serializable {
private int normalField;
private transient String transientField;
}
The transientField will not be serialized, which is important for fields that contain sensitive data or are not serializable.
Question 57: What Is the synchronized
Keyword in Java, and How Does It Ensure Thread Safety? Provide Examples.
How to Respond: Interviewers ask this to assess your knowledge of thread synchronization. Explain that the synchronized
keyword is used to create synchronized blocks or methods for thread safety and provide examples.
Sample Answer: javaCopy code
public synchronized void synchronizedMethod() { // Thread-safe code }
Sample Answer: The synchronized keyword in Java is used to create synchronized blocks or methods, ensuring that only one thread can access them at a time. This prevents race conditions and maintains thread safety. Here's an example of a synchronized method:
public synchronized void synchronizedMethod() {
// Thread-safe code
}
Synchronization is crucial when multiple threads access shared resources or data to avoid concurrent modifications and inconsistencies.
Question 58: What Is the Purpose of the strictfp
Keyword in Java, and When Should It Be Used? Provide Examples.
How to Respond: Interviewers ask this to assess your understanding of the strictfp
keyword. Explain that strictfp
ensures consistent floating-point calculations and provide examples.
Sample Answer: The strictfp keyword in Java ensures consistent floating-point calculations across different platforms by adhering to the IEEE 754 standard. It should be used when precise and consistent floating-point results are required. Here's an example of a method using strictfp:
public strictfp double performCalculation(double x, double y) {
return x / y;
}
strictfp helps maintain consistent behavior for floating-point operations, making it essential for scientific or financial applications.
Question 59: What Are the Key Benefits of Java's Garbage Collection Mechanism, and How Does It Improve Memory Management?
How to Respond: Interviewers ask this to assess your knowledge of garbage collection. Explain the benefits of Java's garbage collection mechanism and how it improves memory management.
Sample Answer: Java's garbage collection mechanism provides several benefits, including automatic memory management, prevention of memory leaks, and simplified resource management. It identifies and collects unreferenced objects, freeing up memory for other tasks. This eliminates the need for manual memory management, which reduces the risk of memory leaks and makes Java more secure and reliable. Garbage collection ensures efficient use of memory and enhances application performance by avoiding memory fragmentation and allowing for better allocation of resources.
Question 60: What Is the Purpose of the this
Keyword in Java, and How Is It Used for Handling Name Conflicts? Provide Examples.
How to Respond: Interviewers ask this to assess your knowledge of the this
keyword. Explain that this
is used to reference the current object and resolve name conflicts, and provide examples.
Sample Answer: In Java, the this keyword is used to reference the current object. It is particularly useful for resolving name conflicts between instance variables and method parameters or for calling constructors within constructors. Here's an example of using this to resolve a name conflict:
public class MyClass {
private int value;
public void setValue(int value) {
this.value = value; // Use 'this' to specify the instance variable
}
}
Using this clarifies the scope of the variable, preventing ambiguity and ensuring that the correct field or method is accessed.
What are the Java Fundamentals?
Java fundamentals are the foundational concepts and principles that form the basis of the Java programming language. They are essential knowledge for anyone starting their journey in Java development. These fundamentals include:
- Syntax and Structure: Understanding the basic syntax, structure, and conventions of the Java language, including packages, classes, and methods.
- Data Types: Knowledge of various data types in Java, such as int, double, String, and their usage in variable declarations.
- Operators: Familiarity with operators like +, -, *, /, and %, used for mathematical and logical operations.
- Control Flow: Mastery of control flow structures like if-else, switch, and loops (for, while, do-while) for making decisions and repeating actions.
- Methods and Functions: Knowing how to declare, invoke, and pass parameters to methods, as well as understanding the concept of return values.
- Object-Oriented Programming (OOP): Grasping the core OOP concepts, including classes, objects, inheritance, polymorphism, and encapsulation.
- Exception Handling: Learning to handle exceptions and errors gracefully using try-catch blocks.
- I/O and File Handling: Understanding input and output operations, including reading and writing to files.
- Collections Framework: Exploring Java's built-in data structures like lists, sets, and maps for efficient data management.
- Multi-Threading: Delving into the world of concurrent programming, managing threads, and synchronizing data.
- Memory Management: Learning about memory allocation, garbage collection, and memory optimization.
- Java Standard Library: Becoming familiar with the vast Java Standard Library, which provides pre-built classes and utilities for various tasks.
What Are the 4 Rules of Java?
The "Four Rules of Simple Design" is a set of principles followed in software development to ensure code simplicity, maintainability, and effectiveness. Although not specific to Java, these rules are valuable in Java development:
- Passes All Tests: Code should pass a comprehensive set of tests, demonstrating its correctness and reliability. In Java, this often means writing and executing JUnit test cases.
- Expresses Intent: Code should be self-explanatory, with clear and meaningful names for variables, classes, and methods. Java code should follow naming conventions for easy understanding.
- No Duplication: Avoid duplication in code by reusing common functionality through inheritance, interfaces, or utility classes. Java promotes code reusability through OOP concepts.
- Fewest Elements: Keep code concise and minimal while achieving the desired functionality. In Java, this means avoiding unnecessary complexity and writing clean, straightforward code.
Adhering to these rules in Java development ensures that your code is maintainable, testable, and easy to understand, contributing to the success of your projects.
Java Fundamentals Cheat Sheet
If you're diving into Java programming or need a quick reference for its fundamentals, this Java Fundamentals Cheat Sheet is your go-to guide. It encapsulates key concepts, syntax, and best practices to help you get started with Java or refresh your memory.
1. Basics of Java
- Java Syntax: Java programs are structured into classes and methods. Understand the basic syntax rules for class and method definitions.
- Data Types: Learn about Java's primitive data types, including int, double, boolean, and more.
- Variables: Declare and initialize variables. Know the scope and lifetime of variables.
2. Operators
- Arithmetic Operators: Use +, -, *, /, and % for mathematical operations.
- Comparison Operators: Employ <, >, ==, and != to compare values.
- Logical Operators: Combine conditions with &&, ||, and ! for logical operations.
3. Control Flow
- if-else Statements: Make decisions using conditional statements.
- Switch Statements: Simplify multiple if-else conditions with switch-case.
- Loops: Create loops with for, while, and do-while for repetitive tasks.
4. Methods and Functions
- Method Declaration: Define methods and specify parameters and return types.
- Method Invocation: Call methods and pass arguments.
- Return Values: Understand how methods return values and how to use them.
5. Object-Oriented Programming (OOP)
- Classes and Objects: Comprehend the concepts of classes and objects, and create instances of classes.
- Inheritance: Implement inheritance to create new classes based on existing ones.
- Polymorphism: Utilize polymorphism to create methods that work with multiple objects.
- Encapsulation: Protect data by encapsulating it within classes and providing access control.
6. Exception Handling
- Try-Catch Blocks: Handle exceptions gracefully using try-catch blocks.
- Throw and Throws: Learn how to throw custom exceptions and declare exceptions using "throws."
7. Input/Output (I/O)
- Reading and Writing Files: Perform file I/O operations using FileReader, FileWriter, and other classes.
- Standard I/O: Use System.in, System.out, and System.err for console input and output.
8. Collections Framework
- Lists, Sets, Maps: Explore Java's built-in data structures and their use cases.
- Iterators: Traverse collections with iterators for efficient data access.
9. Multi-Threading
- Thread Creation: Create and manage threads for concurrent execution.
- Synchronization: Ensure thread safety using synchronization techniques.
- Thread States: Understand thread states, such as NEW, RUNNABLE, BLOCKED, WAITING, and TERMINATED.
10. Memory Management
- Garbage Collection: Learn how Java's garbage collector manages memory.
- Memory Optimization: Optimize memory usage by minimizing object creation.
11. Java Standard Library
- Pre-built Classes: Leverage the extensive Java Standard Library for various tasks, such as string manipulation, date and time operations, and network communication.
This Java Fundamentals Cheat Sheet provides a quick reference for your Java programming journey. Use it to reinforce your understanding of Java basics and write more efficient and readable code.
Java Fundamentals: Data Types
In Java, data types are the building blocks of any program. They define the kind of data that variables can hold. Understanding data types is fundamental for writing Java code. Here are the primary data types in Java:
- Primitive Data Types:
- int: Used for whole numbers, e.g., 5, -42, 0.
- double: Represents decimal numbers, e.g., 3.14, -0.5.
- boolean: Holds true or false values.
- char: Stores single characters, e.g., 'A', '4'.
- byte, short, long, float: Used for various numeric values with different ranges and precision.
- Reference Data Types:
- Objects: Instances of classes.
- Arrays: Ordered collections of elements.
Understanding and using these data types is crucial for Java programming.
What Are the 5 Pillars/ Principles of Java?
Java, as a programming language, is known for its "Write Once, Run Anywhere" philosophy, which is built on five fundamental pillars:
- Simple: Java's syntax and features are designed to be straightforward and easy to understand. This simplicity promotes readability and reduces the likelihood of errors in code.
- Object-Oriented: Java is founded on the principles of object-oriented programming (OOP). Everything in Java is an object, promoting code organization, reusability, and modularity.
- Platform-Independent: Java programs are compiled into bytecode, which can run on any platform with a compatible Java Virtual Machine (JVM). This platform independence makes Java highly portable.
- Secure: Java offers robust security features to protect against unauthorized access and potential threats. It includes features like the Java Security Manager and byte code verification.
- High Performance: Java is designed to provide high performance, even in resource-constrained environments. Its Just-In-Time (JIT) compilation and memory management contribute to its efficiency.
These five pillars are the foundation of Java's success as a versatile and widely-used programming language. They enable developers to build secure, efficient, and easily maintainable applications across various platforms.
How to Practice Java Fundamentals for a Job Interview - Step by Step Guide
Preparing for a Java fundamentals interview is crucial to demonstrate your knowledge and secure the job you desire. Here's a step-by-step guide on how to effectively practice and ace your interview:
- Understand the Job Requirements: Carefully read the job description to identify the specific Java fundamentals you need to focus on. This will guide your study.
- Review Core Concepts: Begin with a comprehensive review of fundamental Java concepts, such as data types, variables, operators, and control structures. Ensure you have a solid understanding of these building blocks.
- Object-Oriented Programming (OOP): Dive deep into OOP principles like classes, objects, inheritance, polymorphism, and encapsulation. Be ready to apply these in practical scenarios.
- Exception Handling: Master the art of handling exceptions gracefully using try-catch blocks. Understand when and how to use them.
- I/O Operations: Learn how to perform input/output operations, including reading from and writing to files, as it's a common task in Java applications.
- Collections Framework: Explore Java's collections like lists, sets, and maps. Understand how to manipulate data efficiently using these data structures.
- Multi-Threading: Study the basics of multi-threading and synchronization. Be prepared to answer questions related to thread safety.
- Memory Management: Gain insights into memory management and garbage collection. Know how Java handles memory to avoid memory leaks.
- Java Standard Library: Familiarize yourself with the Java Standard Library. Be ready to use its classes and utilities for various tasks.
- Practice Coding: Put your knowledge into practice by solving Java programming exercises and coding challenges. Websites like LeetCode, HackerRank, and CodeSignal offer Java-specific questions.
- Mock Interviews: Arrange mock interviews with peers or mentors. This helps you get accustomed to interview settings and receive feedback on your performance.
- Review and Reflect: After each practice session, review what you've learned and reflect on your progress. Identify areas where you need further improvement.
Tips for Cracking Your Java Fundamentals Interview
Cracking a Java fundamentals interview is not just about knowledge; it's also about showcasing your skills effectively. Here are some tips to help you succeed:
- Know Your Basics: Ensure you have a strong grasp of core Java fundamentals. These will form the basis of more complex questions.
- Communication Matters: Articulate your thoughts clearly and concisely. Explain your approach when solving coding problems and clarify your reasoning.
- Problem-Solving Skills: Show your problem-solving prowess. Interviewers often present real-world scenarios to assess your ability to address challenges.
- Code Neatly: Write clean, well-structured code during coding exercises. Good coding practices demonstrate your professionalism.
- Ask Questions: If you're unsure about a question or need clarification, don't hesitate to ask the interviewer. It shows your willingness to learn and collaborate.
- Prepare for Behavioral Questions: Expect questions about your experiences and how you handle situations. Use the STAR method (Situation, Task, Action, Result) to frame your responses.
- Stay Updated: Keep abreast of the latest Java trends, tools, and best practices. This demonstrates your commitment to staying relevant in the field.
- Stay Calm: Interviews can be nerve-wracking, but staying calm and composed is essential. Practice mindfulness techniques to manage stress.
- Dress Appropriately: Dress professionally, even for virtual interviews. First impressions count.
- Follow Up: Send a thank-you email after the interview to express your appreciation and reiterate your interest in the position.
By following this step-by-step guide and adhering to these tips, you'll be well-prepared to excel in your Java fundamentals interview and secure your desired job.